2019.04.30
放射状模様
はじめに
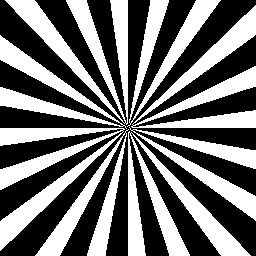
Javaソースコード
Pattern_Radiation01.java
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123
import java.awt.image.BufferedImage; import java.io.File; import javax.imageio.ImageIO; import java.io.IOException; public class Pattern_Radiation01 { // Math.atan2メソッドを利用し、角度の戻り値を0~2πにしたもの private static double atan3( double y, double x ) { // yが0以上の場合 if ( 0.0 <= y ) return Math.atan2( y, x ); // yが0未満の場合 return Math.atan2( y, x ) + 2.0 * Math.PI; } // メイン public static void main( String[] args ) { // 変数宣言 int w, h; // 画像サイズ int l_num; // 本数 double l_deg; // 線の角度 double w_deg; // 白部分の角度 String outname; // 出力ファイル名 BufferedImage img = null; // 画像格納クラス // 入力した引数が5以上かを調べる if ( 5 > args.length ) { // 入力した引数が5未満の場合、使用方法を表示する System.out.println( "Pattern_Radiation01 [PNG名] [幅] [高] [線の本数] [線の角度]" ); return; } try { // 引数を変換し、画像の幅と高さをwとhに代入 w = Integer.valueOf( args[ 1 ] ); h = Integer.valueOf( args[ 2 ] ); // 引数を変換し、線の本数l_numに代入 l_num = Integer.valueOf( args[ 3 ] ); if ( 1 > l_num ) { System.out.println( "線の本数に1以上を指定!" ); return; } // 引数を変換し、線の角度l_degに代入 l_deg = Double.valueOf( args[ 4 ] ); if ( 0.0 >= l_deg ) { System.out.println( "線の角度に0.0より大きい値を指定!" ); return; } } catch( NumberFormatException ne ) { System.out.println( "引数が不正です" ); return; } // 出力PNG名をoutnameに代入(拡張子".png"省略なし) outname = args[ 0 ]; // 新しい画像を作成 // 24ビットカラーの画像を作成 try { img = new BufferedImage( w, h, BufferedImage.TYPE_INT_RGB ); } catch ( Exception e ) { // 画像作成に失敗したときの処理 e.printStackTrace(); return; } // 放射状模様 画像作成 int x, y; int color, r, g, b; // 計算した色 double mx, my; // 画像の中心の座標 double deg, rad; // (mx,my)を原点とした(x,y)の角度 // 画像の中心座標を計算 mx = (double)( w - 1 ) / 2.0; my = (double)( h - 1 ) / 2.0; // 白部分の角度 w_deg = ( 360.0 - (double)l_num * l_deg ) / (double)l_num; // 画像作成 for ( y = 0; y < h; ++ y ) { for ( x = 0; x < w; ++ x ) { // 角度(ラジアン)を計算 rad = atan3( (double)y - my, (double)x - my ); // ラジアンを度に変換 deg = rad * 180.0 / Math.PI; // 判定式 if ( l_deg >= ( deg % ( l_deg + w_deg ) ) ) r = g = b = 0; else r = g = b = 255; // rgbを合成 color = ( r << 16 ) + ( g << 8 ) + b; // 色を設定 img.setRGB( x, y, color ); } } try { // imgをoutname(出力PNG)に保存 boolean result; result = ImageIO.write( img, "PNG", new File( outname ) ); } catch ( Exception e ) { // outname(出力PNG)の保存に失敗したときの処理 e.printStackTrace(); return; } // 正常に終了 System.out.println( "正常に終了しました" ); } }
コンパイル ソースコードが「ANSI」の場合
C:\talavax\javasample>javac -encoding sjis Pattern_Radiation01.java
コンパイル ソースコードが「UTF-8」の場合
C:\talavax\javasample>javac Pattern_Radiation01.java
実行
C:\talavax\javasample>java Pattern_Radiation01 pattern_radiation01.png 256 256 20 10
Javaソースコードの解説
001 002 003 004
import java.awt.image.BufferedImage; import java.io.File; import javax.imageio.ImageIO; import java.io.IOException;
Javaのクラスライブラリの中から「java.awt.image.BufferedImage」と「java.io.File」と「javax.imageio.ImageIO」と「java.io.IOException」というパッケージにあるクラスを、このプログラム内で使うために記述します。この記述により、BufferedImageクラスとImageIOクラスが利用できるようになります。
006
public class Pattern_Radiation01 {
クラス名を、Pattern_Radiation01としています。
007 008 009 010 011 012 013 014 015 016
// Math.atan2メソッドを利用し、角度の戻り値を0~2πにしたもの private static double atan3( double y, double x ) { // yが0以上の場合 if ( 0.0 <= y ) return Math.atan2( y, x ); // yが0未満の場合 return Math.atan2( y, x ) + 2.0 * Math.PI; }
角度を求める方法は、以下の記事を参考にしてください。
019 020
// メイン public static void main( String[] args ) {
021 022 023 024 025 026 027
// 変数宣言 int w, h; // 画像サイズ int l_num; // 本数 double l_deg; // 線の角度 double w_deg; // 白部分の角度 String outname; // 出力ファイル名 BufferedImage img = null; // 画像格納クラス
029 030 031 032 033 034 035
// 入力した引数が5以上かを調べる if ( 5 > args.length ) { // 入力した引数が5未満の場合、使用方法を表示する System.out.println( "Pattern_Radiation01 [PNG名] [幅] [高] [線の本数] [線の角度]" ); return; }
037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062
try { // 引数を変換し、画像の幅と高さをwとhに代入 w = Integer.valueOf( args[ 1 ] ); h = Integer.valueOf( args[ 2 ] ); // 引数を変換し、線の本数l_numに代入 l_num = Integer.valueOf( args[ 3 ] ); if ( 1 > l_num ) { System.out.println( "線の本数に1以上を指定!" ); return; } // 引数を変換し、線の角度l_degに代入 l_deg = Double.valueOf( args[ 4 ] ); if ( 0.0 >= l_deg ) { System.out.println( "線の角度に0.0より大きい値を指定!" ); return; } } catch( NumberFormatException ne ) { System.out.println( "引数が不正です" ); return; } // 出力PNG名をoutnameに代入(拡張子".png"省略なし) outname = args[ 0 ];
与えられた引数をそれぞれ、作成する画像の幅/高さ、線の本数/角度、出力PNG名を格納する変数に代入しています。画像の幅/高さ/線の本数は、Integerクラスを使ってint型に変換しています。線の角度は、Doubleクラスを使ってdouble型に変換しています。
064 065 066 067 068 069 070 071 072 073
// 新しい画像を作成 // 24ビットカラーの画像を作成 try { img = new BufferedImage( w, h, BufferedImage.TYPE_INT_RGB ); } catch ( Exception e ) { // 画像作成に失敗したときの処理 e.printStackTrace(); return; }
BufferedImageコンストラクタ
BufferedImage( int width, int height, int imageType )
・新しい BufferedImage を構築します。 パラメータ width : 構築する画像の横ピクセル height : 構築する画像の縦ピクセル imageType : 構築する画像のイメージ形式
075 076 077 078 079
// 放射状模様 画像作成 int x, y; int color, r, g, b; // 計算した色 double mx, my; // 画像の中心の座標 double deg, rad; // (mx,my)を原点とした(x,y)の角度
081 082 083
// 画像の中心座標を計算 mx = (double)( w - 1 ) / 2.0; my = (double)( h - 1 ) / 2.0;
画像の中心座標を求める方法は、以下の記事を参考にしてください。
085 086
// 白部分の角度 w_deg = ( 360.0 - (double)l_num * l_deg ) / (double)l_num;
088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108
// 画像作成 for ( y = 0; y < h; ++ y ) { for ( x = 0; x < w; ++ x ) { // 角度(ラジアン)を計算 rad = atan3( (double)y - my, (double)x - my ); // ラジアンを度に変換 deg = rad * 180.0 / Math.PI; // 判定式 if ( l_deg >= ( deg % ( l_deg + w_deg ) ) ) r = g = b = 0; else r = g = b = 255; // rgbを合成 color = ( r << 16 ) + ( g << 8 ) + b; // 色を設定 img.setRGB( x, y, color ); } }
110 111 112 113 114 115 116 117 118
try { // imgをoutname(出力PNG)に保存 boolean result; result = ImageIO.write( img, "PNG", new File( outname ) ); } catch ( Exception e ) { // outname(出力PNG)の保存に失敗したときの処理 e.printStackTrace(); return; }
BufferedImageクラスのimgのメモリ内のデータを、出力PNG名の変数(outname)に格納されているファイル名で保存します。この場合は、PNGファイル名が不正であったり、保存先のHDDなどが存在していなかったり、空き容量が少ないなどが原因で処理が失敗する可能性があります。
120 121
// 正常に終了 System.out.println( "正常に終了しました" );
全ての処理が正常終了すると、ここまで処理が実行されます。
以上です。
放射状模様に関するコンテンツ
「放射状模様」に関係があるコンテンツをまとめています。
関連コンテンツ
一般に使われている画像フォーマットには、いろいろな種類があります。画像フォーマットBMP、JPEG、PNG、GIF、TIFFの特徴を知ってますか?
2015.11.29